Lists are like arrays.
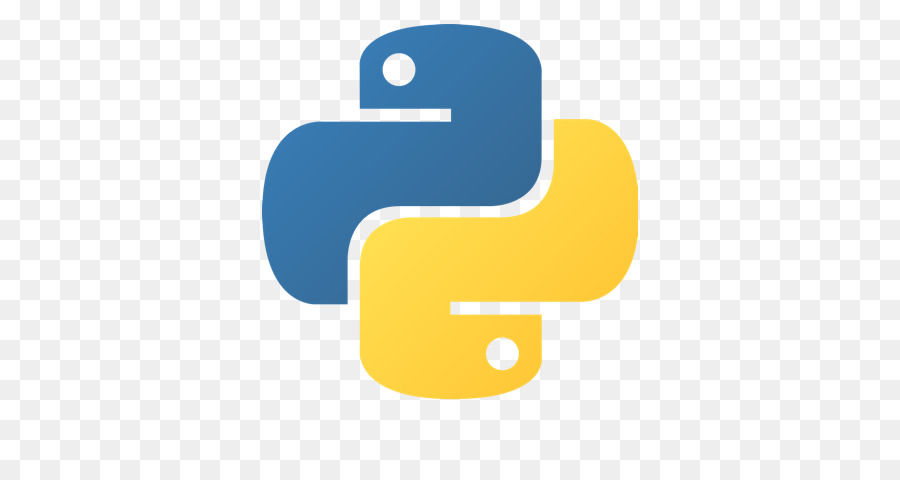
A list can have elements of any data type.
a = [10,20,30,40,50, "ABC", True, 2.09, 3j]
print(a)
The len() is used to get the length of the list.
print(len(a))
The type() gives the data type of the variable.
print(type(a))
The list elements start with index 0.
print(a[0])
-1 can be used to access the last element.
print(a[-1])
When we provide a range, the elements from the number start from the first index till last index - 1 of the range is printed.
print(a[2:7])
When the second index after : is not specified, it means all the elements starting from the start index till the end is printed.
print(a[3:])
When the first index before : is not specified, it means all the elements starting from 0 till the end index -1 is printed.
print(a[:4])
To check whether an element is present in a list or not, we can directly use if statement and verify if an element is present.
if 205 in a:
print(True)
else:
print(False)
The insert() is used to add an element to a particular index.
a.insert(2,350)
print(a)
The append method adds an element to the end of the list
a.append(600)
print(a)
The extend method extends a list using another list.
b = [1.8, 2.8]
a.extend(b)
print(a)
The remove method removes an element from a list.
a.remove(1.8)
print(a)
The pop method removes the last item in a list.
a.pop()
print(a)
Specifying an index within the pop method will remove the item from the corresponding index.
a.pop(2)
print(a)
For loop or while loop can be used to loop through every item in a list.
#looping through a list
for x in a:
print(x)
#looping through index
for i in range(len(a)):
print(a[i])
i = 0
while i < len(a):
print(a[i])
i = i + 1
Using list comprehension, an entire list can be traversed in a single line. The result is a new list.
#list comprehension
print("List Comprehension")
[print(x) for x in a]
The clear method removes all elements in a list.
a.clear()
print(a)
The del key word deletes the entire list.
#delete the list completely
del a
The link to the Github repository is here .