Tuples are data types just like an array. However, the values inside tuples cannot be modified.
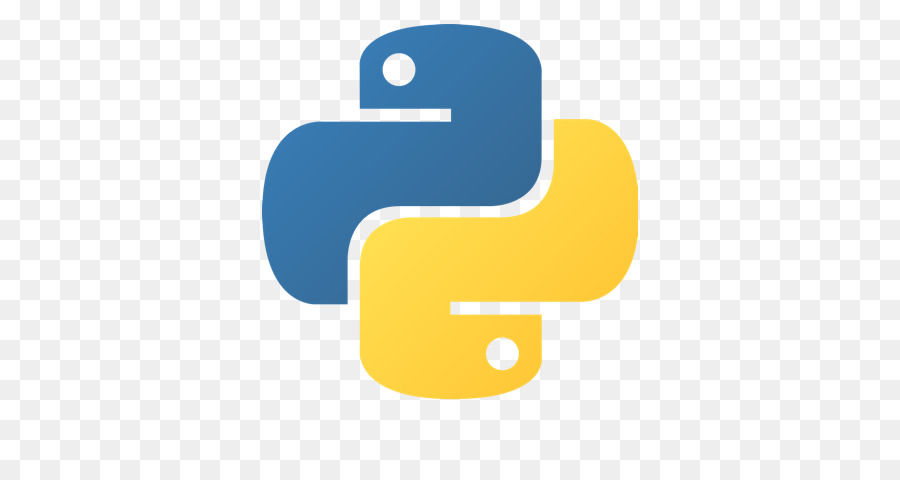
a = ('a',1)
print(a)
print(len(a))
b = (1,2,3)
print(b)
Tuple elements can be accessed using the index.
print(b[0])
print(b[:1])
print(b[0:])
An element can be checked if it is present inside a tuple or not using the if statement.
if 1 in b:
print(True)
else:
print(False)
Depending on the number of elements present in a tuple, it can be unpacked in the following way:
#unpacking tuples
x, y, z = b
print(x, y, z)
A tuple can be traversed using a for loop or a while loop.
for i in b:
print(i)
for i in range(len(b)):
print(b[i])
i = 0
while i < len(b):
print(b[i])
i = i + 1
Two tuples can be concatenated to get a new tuple using the + operator.
c = a + b
print(c)
The link to the Github repository is here .